Automating Tracetest Tests via TypeScript or JavaScript
.avif)

Learn how to programmatically run trace-based tests from JavaScript or TypeScript, allowing for automated setup and execution within code flows!
Table of Contents
A customer recently asked for the ability to run Tracetest tests programmatically from JavaScript or TypeScript so they could execute setup steps directly in code, then fire off a trace-based test. The need to execute setup steps, or to automated the running of Tracetest as part of other automation sequences are common patterns, so we have released a new [npm package called `@tracetest/client`](https://www.npmjs.com/package/@tracetest/client) that allows you to programmatically add and run Tracetest tests.
> *Want a “codeless” way to write your setup or tear down steps? You can define steps using our test suite capability. Read “[Setup and Teardown of Tracetest Tests with Test Suites](https://tracetest.io/blog/setup-and-teardown-of-tracetest-tests-with-test-suites)”.*
## What is Trace-based Testing and Why You Should Care?
Before we dive in, let me answer the first question: **“What the heck is Tracetest?”**
It’s an observability-enabled testing tool for Cloud Native architectures. Distributed tracing was introduced to empower SREs to troubleshot the complex, interconnected systems being used in todays microservice and FaaS-based architectures. Tracetest leverages these distributed traces as part of testing, providing you with better visibility and testability via a technique known as trace-based testing.
## Learning by doing!
This guide will focus on how to use the new NPM package by showing a real example running against a publicly accessible test environment. This example solves a common testing problem, externally setting up data in the test environment before running a test. The scenario is:
```bash
As a user,
I want to be able to test
our delete Pokemon endpoint
but need to add a Pokemon
via an external POST call first
```
We will explain the system under test, further discuss the test scenario and explain code for key sections, then have you clone the repo and run the Typescript code yourself.
## The Test Environment
We are going to use a pre-deployed version of our Pokeshop demo which is sending its telemetry information to Jaeger. This instance is publicly available so you can run tests against it. You can even view the UI at [https://demo-pokeshop.tracetest.io/](https://demo-pokeshop.tracetest.io/). The code for this [Pokeshop Demo is available here](https://github.com/kubeshop/pokeshop).
## The Test Scenario
This is the scenario we are going to execute via TypeScript against our Pokeshop test environment:
Add an entity via a POST to a REST endpoint. For this example, we are adding a Pokemon programmatically as a setup step using the Node.js built-in `fetch` command.
Run a Tracetest `delete-pokemon` test using the @tracetest/client [NPM package](https://www.npmjs.com/package/@tracetest/client). We will:
- Authenticate to app.tracetest.io.
- Upsert a delete-pokemon Tracetest test.
- Run the delete-pokemon Tracetest test.
- Print a summary.
The code to execute this scenario is contained in [the `delete_test.ts` file](https://github.com/kubeshop/tracetest/blob/main/examples/setup-of-tracetest-tests/delete_test.ts) from a repo which we will clone and run locally further in this article. First, let’s discuss the code for the key sections that are utilizing the @tracetest/client [NPM package](https://www.npmjs.com/package/@tracetest/client).
## Breakdown and Explanation of Key Sections
### Instantiate Tracetest & Authenticate to [app.tracetest.io](http://app.tracetest.io) with an API Token
This code does a few things:
- Instantiates the module.
- Defines an API token - read more about tokens in the included comment.
- Defines the tracetest object using the `await Tracetest(TRACETEST_API_TOKEN)` call. We will use this `tracetest` object in future calls to interact with Tracetest.
```javascript
import Tracetest from '@tracetest/client';
// To use the @tracetest/client, you must have a token for the environment. This is created on the Settings
// page under Tokens by the administrator for the environment. The token below has been given the 'engineer'
// role in the pokeshop-demo env in the tracetest-demo org so you can create and run tests in this environment.
// Want to read more about setting up tokens? https://docs.tracetest.io/concepts/environment-tokens
const TRACETEST_API_TOKEN = 'tttoken_4fea89f6e7fa1500';
console.log('Lets use the TRACETEST_API_TOKEN to authenticate the @tracetest/client module...')
const tracetest = await Tracetest(TRACETEST_API_TOKEN);
```
### Upsert the delete-pokemon Test
Open the delete_pokemon.yaml file which contains the delete-pokemon test and upsert it to the current environment. This is similar to running the Tracetest CLI command: `tracetest apply test -f delete_pokemon.yaml`.
```javascript
// Lets pull in the delete-pokemon test from a file
let deleteTest = fs.readFileSync('delete_pokemon.yaml', 'utf-8');
const test = await tracetest.newTest(deleteTest);
```
This command returned a `test` object which is used in the next step.
### Run the delete-pokemon Tracetest Test
Run the test while passing variables. The variable in this example contains the id of the Pokemon to be deleted in the delete-pokemon test.
```javascript
// run deletes pokemon test
console.log('Running the delete-pokemon test...');
const run = await tracetest.runTest(test, { variables: getVariables(String(pokemonId)) });
run.wait();
```
### Print a Summary of the Test Result
Prints a summary of the test result.
```javascript
console.log(await tracetest.getSummary());
```
## Run the TypeScript Script
Enough talking… lets see this TypeScript script run locally. You will need Node and npm installed - see the [Downloading and installing Node.js and npm](https://docs.npmjs.com/downloading-and-installing-node-js-and-npm) instructions. You should be able to run both `node -v` and `npm -v` before trying this example.
First, clone the repo:
```bash
git clone https://github.com/kubeshop/tracetest
cd tracetest/examples/setup-of-tracetest-tests
```
Open the `delete_test.ts` file in this directory. You will see the key sections discussed earlier in this article. To run the example, you only need to execute these commands:
```bash
npm install typescript -g
npm i @tracetest/client
npm i # to install dev dependencies
npm run build
npm start
```
Upon running the program, you will see:
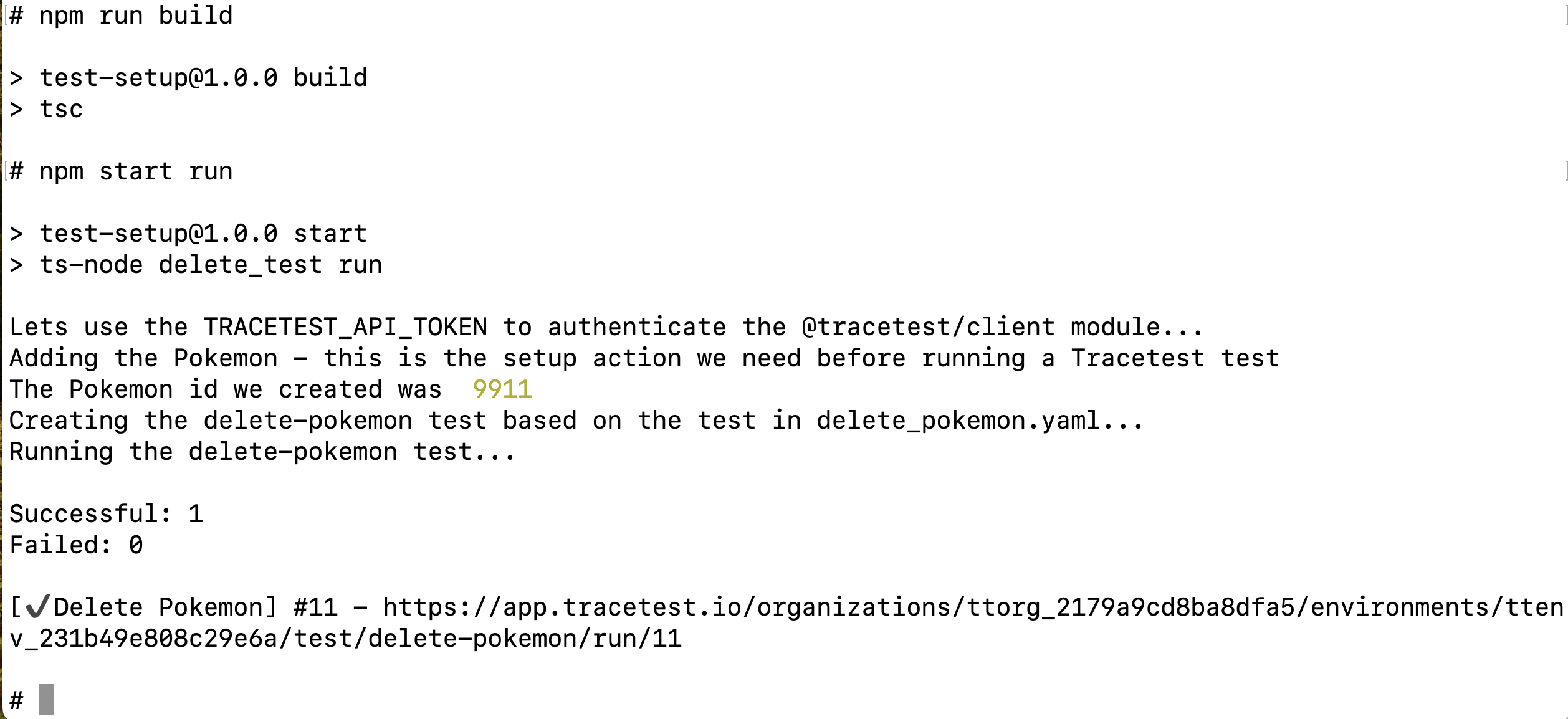
Great! You successfully ran `delete_test.ts` . The `tracetest.getSummary()` shows a brief summary of the test and provides a link to the test run on app.tracetest.io. To see the test response, you will need to be a member of this “Pokeshop Demo” environment. To join, click this link:
➡︎ [**Join the Pokeshop Demo**](https://app.tracetest.io/organizations/ttorg_2179a9cd8ba8dfa5/invites/invite_760904a64b4b9dc9/accept) ⬅︎
You will be prompted to sign in with either your GitHub or Google account. Once logged in, click the “Join organization” button to get access to the Pokeshop demo environment.
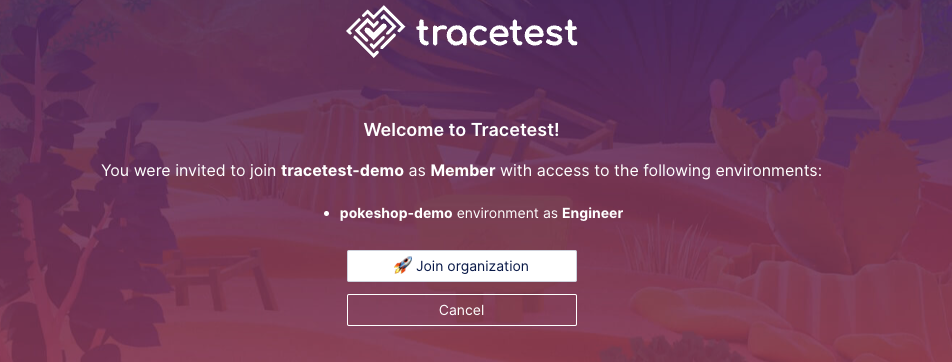
Great - you are now a member of the organization with access to the Pokeshop demo environment!
You can then follow the link to the test run, click on the “Test” tab and see the results for the `delete-pokemon` test.
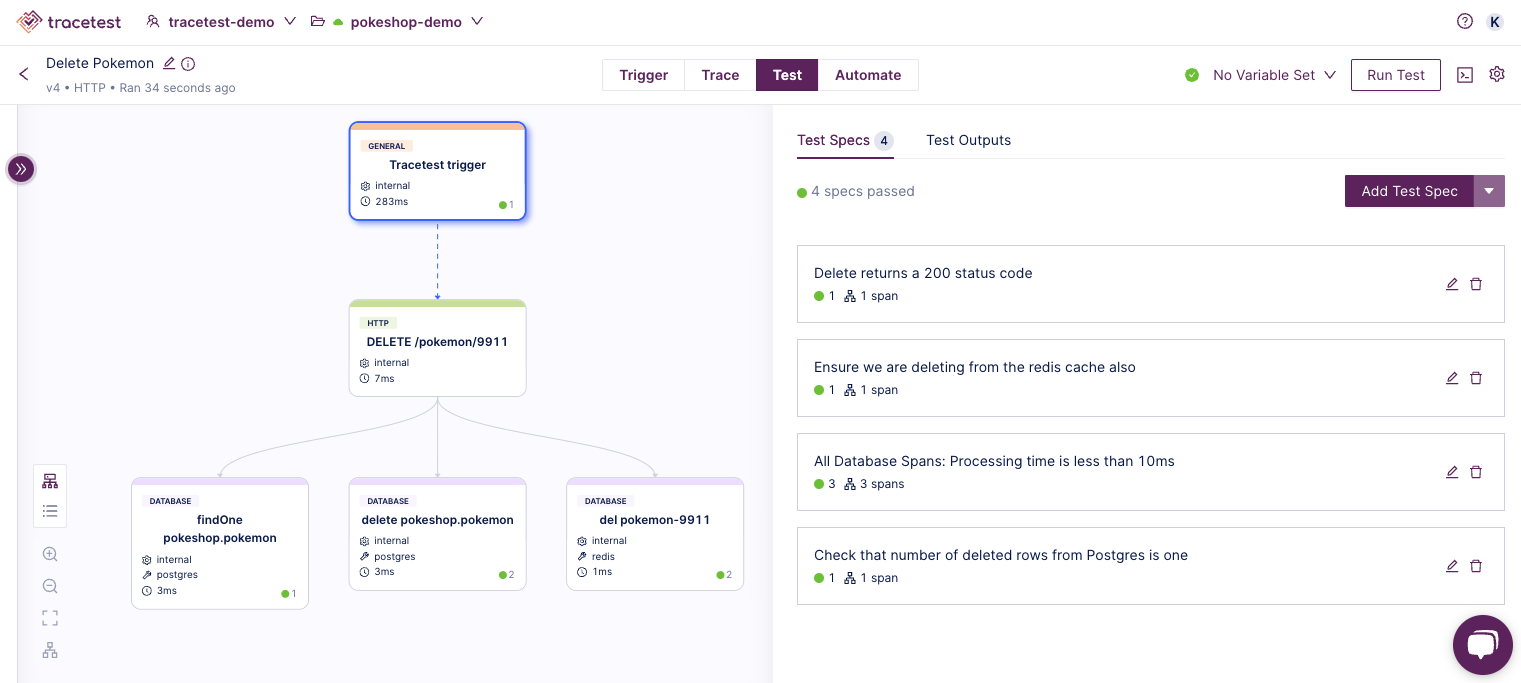
Congrats - you successfully ran the example. Want to look at another example? Checkout the [Programmatically triggered trace-based tests using Tracetest and TypeScript](https://docs.tracetest.io/tools-and-integrations/typescript) quick start guide.
## What’s next?
Now that you have seen how to run Tracetest tests via your own TypeScript code, you are now ready to create your own tests and embed them in custom flows!
To use Tracetest to test your own environment, follow these steps:
- Create a new [organization](https://docs.tracetest.io/concepts/organizations) and [environment](https://docs.tracetest.io/concepts/environments).
- Use the environment setup wizard to enable your Tracetest environment to run tests against and collect distributed trace information from your system under test.
- Start creating tests!
Have questions? The devs and I hang out in the [Tracetest Slack channel](https://dub.sh/tracetest-community) - join, ask, and we will answer!